Overview
The Real App is an enhanced desktop address book APP. The enhanced application enables the real estate agents to store large amount of customer contacts, together with property information; provide fleet retrieval commands among tons of property information; and utilize storage better to provide reminder, archive and corresponding features. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
This section shows a summary of my coding, documentation, and other helpful contributions to the team project. |
-
Major enhancement: implement the pin/unpin feature
-
What it does:
-
The pin command allows the user to put some contacts from the normal contact list to the topped pin list. The
pinselect
command will select some contact in the pin list, which will displays all the contact and property information, as well as the address location of the associated property on the Google Maps™ browser panel.
-
-
Justification:
-
This feature improves the product significantly because a user can put important contacts into the topped list, which can save much time for searching.
-
-
Highlights:
-
This enhancement does not affect commands that will not touch pin list. Since the pin list is apart from the original one and works as a fast and detailed look-up manual towards someone the agent currently working with or extremely important contacts. The most challenging part is about the connection requires to build between the brand-new storage for pin list and the other parts. Another point is the UI part displayed in the main window.
-
It required an in-depth analysis of design alternatives.
-
-
-
Minor enhancement: Apply
undo
redo
command to the new commands. So user can directly useundo
to reverse the changes and useredo
to reverse the most recent changes made byundo
command. -
Code contributed: [Project Code Dashboard]
-
Other contributions:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Pinning a contact : pin
Pins a contact.
Limited to a maximum of 5 contacts, these contacts will always be showing in a pin list at the top of the side panel.
Format: pin INDEX
Pinned contacts must be unpinned before any other commands can be performed, except for |
Examples:
-
list
pin 2
Pins the 2nd contact in the contact book. -
search James
pin 1
Pins the 1st contact in the results of thesearch
command. -
search seller
pin 2
Selects the 2nd contact in the results of thesearch
command.
Screenshots for 1st example:
-
Enter
list
:

-
Main contact list is displayed:
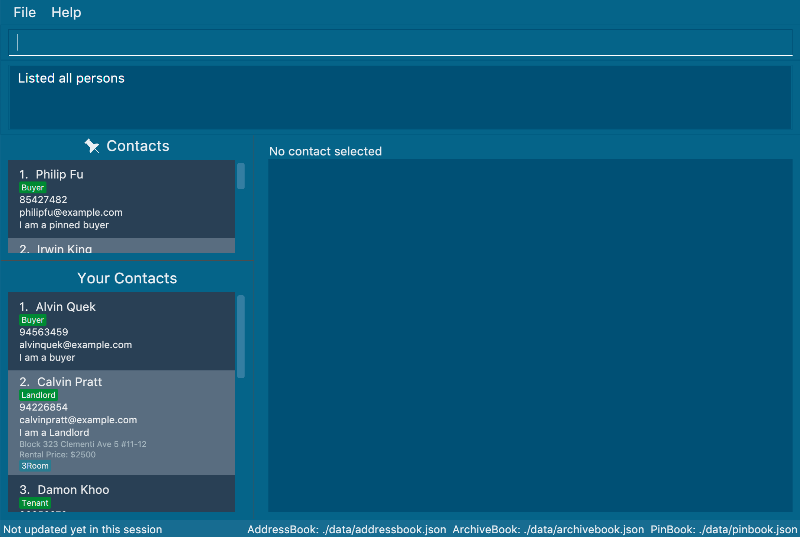
-
Enter
pin 2
:
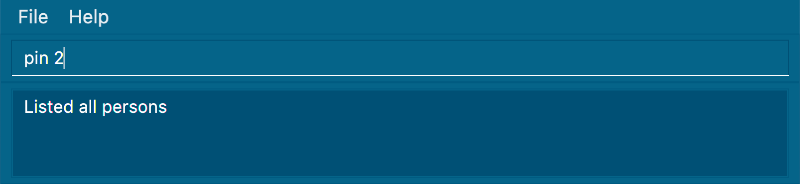
-
2nd contact has been successfully pinned:
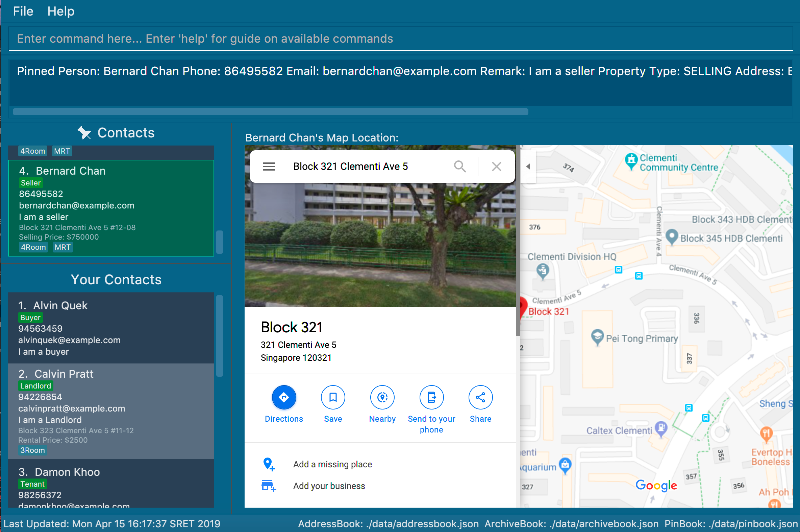
Unpinning a contact : unpin
Unpins a pinned contact.
Format: unpin INDEX
Example:
-
unpin 1
Unpins the 1st contact in the pin list.
Selecting a pinned contact : pinselect
Select a pinned contact identified by the index number used in the displayed pin list.
Format: pinselect INDEX
Go to [GoogleMaps] for more details on the Google Maps™ display. |
Examples:
-
pinselect 3
Selects the 3rd contact in the pin book.
Screenshots for the example:
-
Enter
pinselect 3
:

-
3rd contact in the pin list has been successfully selected:
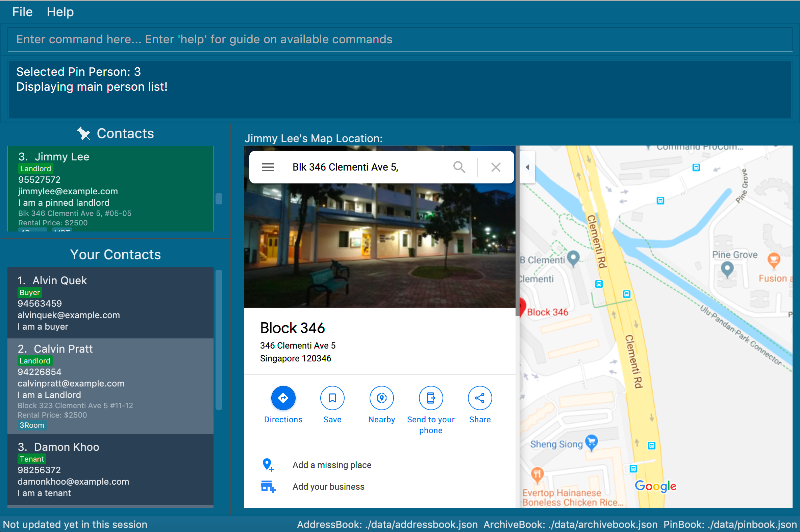
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
*
Pin/Unpin feature
This feature allows users to move person between contact list and pin list. To use this feature, the user need to enter pin
or unpin
command, with the INDEX
(in the contact list and pin list) of the contact to be pinned/unpinned.
-
pin 2
The command above put the 2nd contact in the contact list to the topped pin list.
-
unpin 1
The command above put the 1st contact in the pin list back to the contact list.
What’s more, it also allow users to select some contact in the pin list and display address location on the Google Maps™ browser window. To use this feature, the users need to enter pinselect
command, with the INDEX
(in the pin list) of the contact to be selected.
-
pinselect 3
The command above will select the 3rd contact in the pin list and the address location is displayed on the Google maps™ browser window.
Current Implementation
This section explains the implementation of all pin related features.
Pin/Unpin
The following sequence diagram shows how the pin operation works:
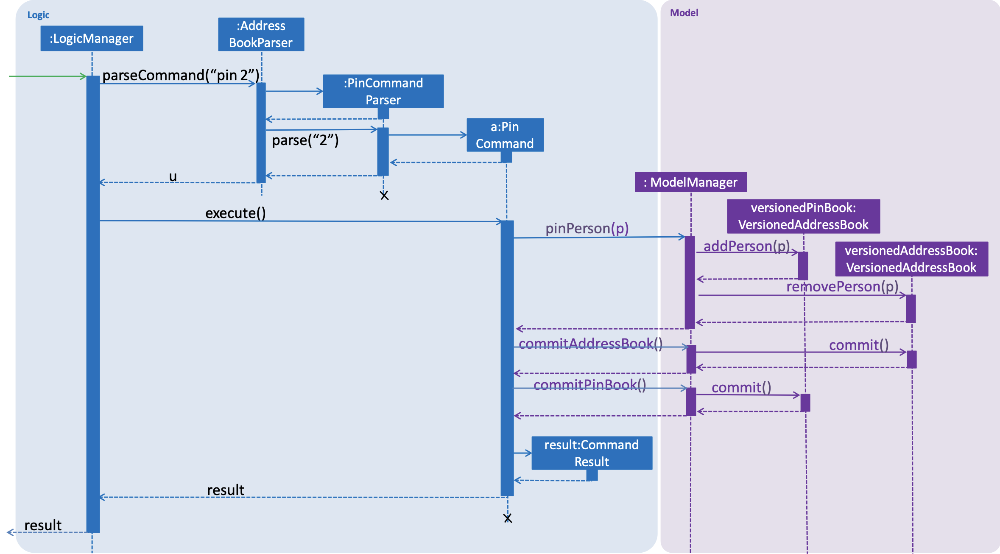
-
The command is recognized by
parserCommand()
and anPinCommandParser
is created, then aPinCommand
object is created with the parsed index. -
The command object is then executed by
Logic
andpinPerson
is called from thePinCommand
object. ThenpinPerson
will callModelManager
to create twoVersionedAddressBook
object:versionedPinBook
andversionedAddressBook
. -
The
versionedPinBook
calladdPerson()
and theversionedAddressBook
callremovePerson()
. -
Both objects will call
commit()
after execution.
The unpin
command does the opposite — it calls removePerson()
of the versionedPinBook
and addPerson()
of the versionedAddressBook
instead.
PinSelect
The pinselect
command is implemented the same as select
.
When pin
and unpin
are performed, the pinned/unpinned contact is being selected automatically.
Design Considerations
Aspect: How pin & unpin executes
-
Alternative 1 (current choice): Saves the entire address book and pin book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2 (current choice): Commands excepts
pin
,unpin
,pinselect
command doesn’t work on the pinned person.-
Pros: Easy to implement.
-
Cons: Users must filter pin list contacts' information through eyes.
-
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire address book and pin book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
Use case: Pin contact
MSS
-
User requests to list contacts.
-
TheRealApp shows a list of contacts.
-
User requests to pin a specific contact in the list.
-
TheRealApp pins the contact.
Use case ends.
Extensions
-
2a. The contact list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. TheRealApp shows an error message.
Use case resumes at step 3.
-
-
3b. There are already 5 contacts in the pinned list.
-
3b1. TheRealApp shows an error message.
Use case ends.
-
Use case: Unpin contact
MSS
-
User requests to unpin a specific pinned contact in the pinned list.
-
TheRealApp unpins the contact.
Use case ends.
Extensions
-
1a. The pinned list is empty.
Use case ends.
-
1b. The given index is invalid.
-
1b1. TheRealApp shows an error message.
Use case resumes at step 1.
-
-
1c. The list displayed is invalid.
-
1c1. TheRealApp shows an error message.
-
1c2. User requests for the valid list.
-
1c3. TheRealApp displays the requested list.
Use case resumes at step 1.
-
Use case: select pinned contact
MSS
-
User requests to select an pinned contact.
-
TheRealApp selects the contact and shows the information of the pinned contact.
Use case ends.
Extensions
-
1a. The given index is invalid.
-
1a1. TheRealApp shows an error message.
Use case resumes at step 1.
-
Pinning a person
-
Pinning a person from contact list to pin list.
-
Prerequisite: There are multiple persons in the contact list.
-
Test case:
pin 3
Expected: Third contact in the contact list is put to the pin list. Address location of the pinned contact is displayed on the Google Maps™ window panel(if applicable). -
Test case:
pin 0
Expected: No person is pinned. Error details shown in the status message. Status bar remains the same. -
Other incorrect unpin commands to try:
pin
,pin x
(where x is larger than the contact list size) Expected: Similar to previous.
-
Unpinning a person
-
Unpinning a person from pin list to contact list.
-
Prerequisite: There are multiple persons in the pin list.
-
Test case:
unpin 1
Expected: First contact in the pin list is put back to the contact list. Address location of the unpinned contact is displayed on the Google Maps™ window panel(if applicable). -
Test case:
unpin 0
Expected: No person is unpinned. Error details shown in the status message. Status bar remains the same. -
Other incorrect unpin commands to try:
unpin
,unpin x
(where x is larger than the pin list size) Expected: Similar to previous.
-
Selecting a person in the pin list
-
Selecting a person in the pin list
-
Prerequisite: There are multiple persons in the pin list.
-
Test case:
pinselect 2
Expected: Second contact in the pin list is selected. Address location of the selected contact is displayed on the Google Maps™ window panel(if applicable). -
Test case:
pinselect 0
Expected: No person is selected. Error details shown in the status message. Status bar remains the same. -
Other incorrect pinselect commands to try:
pinselect
,pinselect x
(where x is larger than the pin list size) Expected: Similar to previous.
-